By default, cocos2d-x applies this technique for the textures we use in our games, but there are situations where we want to disable this effect. For example, for games with pixelated aesthetics.
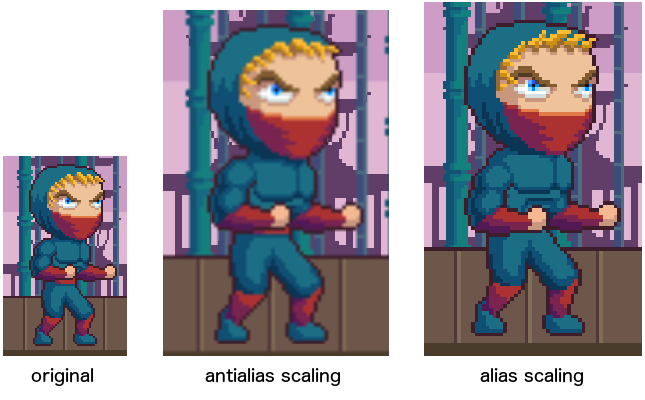
The way to disable anti alias is calling method setAliasTexParameters() on the used textures. I don’t know if there is a simple global manner to do it for the whole game, so I call to setAliasTexParameters() for every texture I use. Below it’s shown how to do it for different elements of the game.
Sprite
Code: Select all
auto sprite = Sprite::create("image.png");
sprite->getTexture()->setAliasTexParameters();
For the sprite atlas (.plist files) we have several ways to access textures. For example:
Get the texture through a sprite
We can access a texture from an existing sprite that uses it. When we set the alias params to the texture, this affects to all sprites that use the same texture. So it’s only neccesary to do it one time for the whole atlas:
Code: Select all
auto sprite = Sprite::createWithSpriteFrameName("sprite_name");
sprite->getTexture()->setAliasTexParameters();
This method loads a texture without smoothing.
Code: Select all
Texture2D* loadAliasTexture(const std::string &textureFile) {
auto img = new Image();
img->initWithImageFile(textureFile);
auto tex = new Texture2D();
tex->initWithImage(img);
tex->setAliasTexParameters();
return tex;
}
Code: Select all
Texture2D* tex = loadAliasTexture("images.png");
SpriteFrameCache::getInstance()->addSpriteFramesWithFile("imgages.plist", tex);
Code: Select all
auto label = Label::createWithBMFont("font.fnt", "Label text");
label->getFontAtlas()->getTexture(0)->setAliasTexParameters();
Original Article